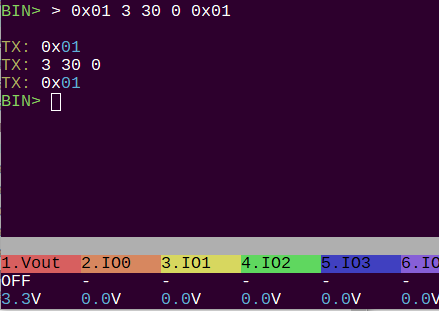
Commands may now have trailing argument bytes, determined in the global_command_struct.
static const struct _binmode_global_struct global_commands[]={
[BM_RESET]={&binmode_reset,0},
[BM_POWER_EN]={&binmode_psu_enable,4},
[BM_POWER_DIS]={&psu_disable,0},
[BM_PULLUP_EN]={&pullup_enable,0},
[BM_PULLUP_DIS]={&pullup_disable,0},
//[BM_AUX]={&auxio_input_handler},
//[BM_ADC]={&adc_measure_single},
};
PSU enable has four argument bytes:
0x01 <volts integer> <volts decimal (/100)> <current ma> <override current limit (bool)>
0x01 3 30 0 1
This enables the PSU with 3.3volts, 0mA, current limit override.
I really like the idea of plain int values in the protocol. Lots of times (old bus pirate, SUMP LA protocol) you need to calculate the register values, which are drastically different on different hardware and not very portable.
Iāve done some really ugly hacks to get old protocols working on new hardware, and this prevents that nonsense. Will do the same with PWM/freq/ADC values, maybe with second command with direct register/value access.
Modes list
Current command 0x05 (these will change) gives a ;
delimited list of plain text mode names. Many that should be simple comma instead?
For the āchange modeā command, I think it would be nice to send these strings back so weāre no longer tied to any fixed numbering of the protocols that might drift over time and cause scripts to break. If we change the name of a mode/protocol, itās easy enough to add a backwards compatible check in binmode.
Error and confirmation
The old binary mode is a jumble of commands that respond or donāt respond. Iām leaning towards a dumb āno-response even on errorā mode, with a command to enable a verbose mode. The default isnāt as important as consistent use of success/fail when configured.
Hereās a test firmware, Iāve also pushed to the binmode branch:
bus_pirate5_rev10-binmode.zip (174.7 KB)